Evolving an i18n Solution: From Astro to Vue.js
· 15m ·
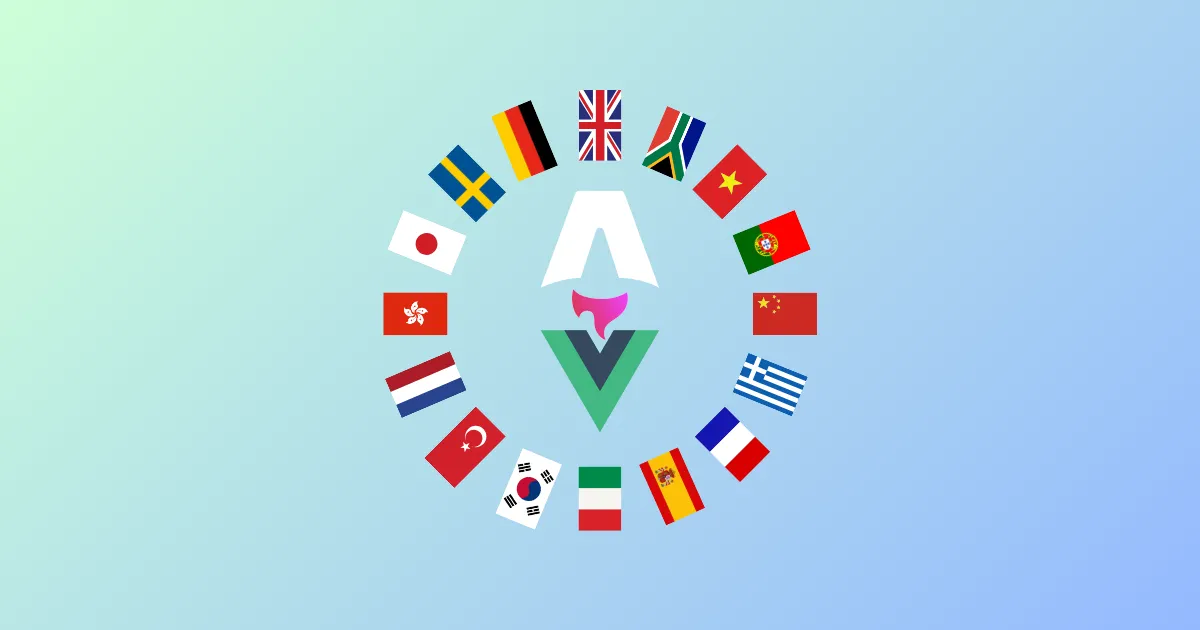
Introduction
When building multilingual web applications, developers often start with simple translation files and basic language switching. However, as applications grow in complexity, this approach quickly reveals its limitations. Missing translations can cause runtime errors, incorrect language paths can break navigation, and maintaining type consistency across multiple languages becomes increasingly challenging. These issues become particularly evident when working with frameworks like Astro and Vue.js, where the boundary between static and dynamic content requires careful consideration.
During the development of a multilingual documentation site, I encountered these challenges firsthand. The initial implementation used a straightforward key-value translation system, but it became apparent that we needed a more robust solution that could handle complex routing patterns, provide compile-time type checking for translations, and seamlessly integrate with both server-side and client-side rendering.
This article details the evolution of our internationalization (i18n) solution, focusing on achieving type safety through TypeScript’s advanced type system. We’ll explore how combining constant assertions, discriminated unions, and template literal types creates a robust foundation for managing multilingual content. The solution we’ll examine handles several critical aspects that are often overlooked in simpler implementations:
- Type-safe translation keys that prevent accidental usage of non-existent translations
- Intelligent path management that maintains SEO-friendly URLs across language switches
- Graceful fallback mechanisms for missing translations
- Framework-agnostic utilities that work in both Astro’s static context and Vue’s dynamic components
- Complex routing patterns that preserve content hierarchy across languages
For instance, consider a typical scenario where a blog post needs to maintain its URL structure across multiple languages while ensuring all translations exist:
// Without type safety:
translate('blog.post.title') // Could fail at runtime if key doesn't exist
// With our type-safe approach:
translateFrom('it', 'blog.post.title' as TranslationKeys) // Caught at compile-time if invalid
The implementation we’ll explore leverages TypeScript’s type system to catch these issues during development, long before they can affect users. Through careful type definitions and utility functions, we’ve created a system that provides excellent developer experience without sacrificing runtime performance or flexibility.
Let’s dive into the technical details of this implementation, starting with our translation management system and progressing through the various utilities that make it all work together seamlessly.
The Translation Hub: ui.ts
At the heart of any internationalization system lies the translation management structure. While many implementations treat translations as simple key-value pairs, our approach leverages TypeScript’s type system to create a more sophisticated and reliable foundation. The translation hub not only stores our translations but also establishes the type relationships that will guide developers throughout the application.
Understanding the structure of ui.ts
is crucial because it sets up the type constraints that flow through the entire system. We begin by defining our default language as a constant using TypeScript’s as const
assertion. This might seem like a minor detail, but it’s essential for maintaining type safety throughout our application.
Consider the challenges of managing translations in a growing application: new languages being added, translations being updated, and the need to ensure consistency across all language versions. Our structure addresses these challenges through careful type definitions and constant assertions. Let’s examine the implementation:
// src/i18n/ui.ts
// Define the default language as a constant
export const defaultLang = 'en' as const;
// Define available languages with their display names
export const languages = {
en: 'English',
it: 'Italiano',
nl: 'Nederlands',
} as const;
// Create a type for available languages
export type AvailableLanguages = keyof typeof languages;
// Define the UI translations structure
export const ui = {
en: {
'flag': '🇬🇧',
'language': 'English',
'nav.home': 'Home',
'nav.blog': 'Blog',
// ... English translations
},
it: {
'flag': '🇮🇹',
'language': 'Italiano',
'nav.home': 'Inizio',
'nav.blog': 'Blog',
// ... Italian transtations
},
nl: {
'disabled': 'true', // Special flag for incomplete translations
'flag': '🇳🇱',
'language': 'Nederlands',
// ... Dutch translations
}
} as const; // Using 'as const' for type inference
// Derive types from our UI structure
export type UI = typeof ui;
export type Languages = keyof UI;
export type TranslationKeys = keyof typeof ui[typeof defaultLang];
The structure we’ve created serves several crucial purposes that might not be immediately obvious:
-
The
as const
assertion on our objects isn’t just a TypeScript detail - it transforms our translation structure from a loose collection of strings into a precise type definition that TypeScript can use to enforce correctness throughout our application. -
By deriving our types from the actual data structure using
typeof
, we ensure that our types always stay in sync with our actual translations. This prevents the common issue of type definitions diverging from implementation over time. -
The special ‘disabled’ flag in the Dutch translations demonstrates how we can handle partially implemented languages without compromising type safety.
The Engine Room: utils.ts
While the translation hub defines our data structure, the utility functions in utils.ts
provide the machinery that makes everything work together. These utilities handle everything from language detection to route translation, forming the backbone of our internationalization system.
Each utility function is designed to handle a specific aspect of the internationalization process while maintaining type safety. The functions work together to create a cohesive system that handles both simple and complex scenarios. Let’s examine these utilities and understand how they work together:
// src/i18n/utils.ts
import { ui, defaultLang, type Languages, type TranslationKeys } from './ui';
// URL-based language detection
export function getLangFromUrl(url: URL | string): Languages {
// Extract language code from URL path
const [, lang] = (typeof url === 'string' ? url : url.pathname).split('/');
// Type guard to ensure language exists in our UI definitions
return (lang in ui) ? lang as Languages : defaultLang;
}
// Translation function with type safety
export function translateFrom(lang: Languages, key: TranslationKeys): string {
// Optional chaining with nullish coalescing for robust fallback
return ui[lang]?.[key] ?? ui[defaultLang][key] ?? `#${key}#`;
}
// Hook-style translation function
export function useTranslate(lang: Languages) {
return function translate(key: TranslationKeys): string {
return translateFrom(lang, key);
}
}
// Path translation with template support
export function translatePath(
path: string,
targetLang: Languages = defaultLang
): string {
const pathSegments = path.split('/');
// Handle already localized paths
if (pathSegments[1] in ui) {
if (pathSegments[1] === targetLang) {
return path;
}
return populateFromRoute(stripLangFromPath(path), targetLang);
}
// Handle non-localized paths
return targetLang === defaultLang
? path
: populateFromRoute(path, targetLang);
}
// Helper function to strip language prefix
export function stripLangFromPath(path: string): string {
const availableLanguages = Object.keys(ui) as Languages[];
return path
.split('/')
.filter(segment => !availableLanguages.includes(segment as Languages))
.join('/');
}
// Template-based route population
export function populateFromRoute(
path: string,
targetLang: Languages
): string {
const variables: Record<string, string> = {
lang: targetLang,
path: path !== '/' ? path : ''
};
// Find matching route template
for (const [route, template] of Object.entries(routesFromEnToLocalized)) {
if (path.startsWith(route)) {
return substituteTemplate(template, variables);
}
}
return path;
}
// Template variable substitution
export function substituteTemplate(
template: string,
variables: Record<string, string>
): string {
return template.replace(/{{(\w+)}}/g, (_, key) => {
if (variables[key] === undefined) {
console.warn(`Missing template variable: ${key}`);
return `Missing value for template variable: ${key}`;
}
return variables[key];
});
}
These utilities demonstrate several important patterns:
-
The language detection system is designed to be resilient, always falling back to the default language rather than throwing errors. This is crucial for maintaining a stable user experience.
-
The route translation system handles complex path transformations while preserving SEO-friendly URLs. This is particularly important for content-heavy sites where URL structure affects search engine rankings.
-
The template system provides flexibility for complex routing patterns while maintaining type safety. This allows us to handle varied URL structures without compromising reliability.
Type Safety and Error Handling
Type safety in an internationalization system goes beyond preventing simple typing errors. It’s about creating a system that guides developers toward correct usage while catching potential issues before they reach production. Our implementation leverages TypeScript’s type system to provide several layers of protection:
- Language Type Guard: The
getLangFromUrl
function ensures we only work with defined languages:
function isValidLanguage(lang: string): lang is Languages {
return lang in ui;
}
- Translation Key Safety: The
TranslationKeys
type ensures we can only request existing translations:
// This would cause a TypeScript error
translateFrom('en', 'nonexistent.key'); // Error: Argument not assignable to TranslationKeys
These type safety mechanisms work together to create a development experience that catches errors early while providing helpful feedback through IDE integration. Rather than discovering missing translations in production, developers receive immediate feedback during development.
Route Translation System
Route translation in a multilingual application presents unique challenges. URLs need to be both user-friendly and SEO-optimized while maintaining consistent structure across languages. Our route translation system addresses these challenges through a template-based approach that provides flexibility without sacrificing type safety:
export const routesFromEnToLocalized = {
'/posts/{{lang}}/notes': '/{{lang}}/posts/{{lang}}/notes{{path}}',
'/posts/notes': '/{{lang}}/posts/{{lang}}/notes{{path}}',
'/posts': '/{{lang}}/posts/{{lang}}{{path}}',
'/': '/{{lang}}{{path}}',
} as const;
This routing system demonstrates several sophisticated features:
- Simple language prefix addition (‘/about’ → ‘/it/about’)
- Complex path transformations (‘/posts/notes’ → ‘/it/posts/it/notes’)
- Path parameter preservation
- Default language path stripping
- It handles complex content hierarchies where the path structure might differ between languages
- It preserves query parameters and hash fragments during translation
- It maintains SEO-friendly URLs across all supported languages
- It handles edge cases like the root path and missing translations gracefully
This system supports:
Vue.js Integration Considerations
Integrating our type-safe internationalization system with Vue.js presents unique challenges. While the core utilities remain the same, we need to adapt our approach to work within Vue’s reactivity system and component lifecycle. The key difference lies in how we handle URL detection and language changes in a client-side environment:
// Vue component example
<script lang="ts" setup>
// imports...
// Reactive language based on URL
const currentLang = ref(defaultLang);
onMounted(() => {
url = new URL(window.location.href);
currentLang.value = getLangFromUrl(url); // Update currentLang reactively
})
// Watch currentLang for changes and update translations
watchEffect(() => {
url = new URL(window.location.href);
currentLang.value = getLangFromUrl(url); // Update currentLang reactively
translate = useTranslate(currentLang.value as Languages);
});
</script>
// [...]
<template>
// [...]
</template>
The Vue.js integration showcases how our type-safe system can adapt to different frameworks while maintaining its core benefits. The reactive nature of Vue components requires careful consideration of when and how we update our translations, but our type system ensures these updates remain type-safe.
Testing Considerations
Testing an internationalization system requires careful attention to both type safety and runtime behavior. Our testing approach verifies not just the happy path but also edge cases and error conditions:
describe('i18n/utils', () => {
it('should create a translation function', () => {
const t = useTranslate('it');
expect(t('nav.home')).toBe(ui.it['nav.home']); //Replace 'nav.home' with an actual key from your ui object
// Please note the forceful typecasting needed to make it fail and test how it would do it in production.
expect(t('nonexistentKey' as TranslationKeys)).toBe(ui.en['nonexistentKey'] ?? '#nonexistentKey#'); //Should handle missing keys gracefully. Modify based on your desired behavior.
});
});
These tests demonstrate how our type system helps ensure reliability while still allowing us to test edge cases and error conditions. The ability to force type errors in our tests helps ensure our error handling works as expected in production.
Conclusion
Building a robust internationalization system requires careful consideration of both developer experience and runtime behavior. Through our journey of implementing this type-safe i18n solution, we’ve seen how TypeScript’s advanced type system can transform what is traditionally an error-prone aspect of web development into a reliable and maintainable foundation for multilingual applications.
The power of this implementation lies not just in its ability to catch errors at compile time, but in how it guides developers toward correct usage through TypeScript’s type inference and IDE integration. When a developer attempts to use a non-existent translation key or incorrectly structures a route, they receive immediate feedback. This immediate feedback loop dramatically reduces the time spent debugging production issues and ensures consistency across the entire application.
What makes this solution particularly valuable is its adaptability across different rendering contexts. Whether working within Astro’s static site generation or Vue’s dynamic component system, the core utilities remain consistent and reliable. This consistency is crucial for larger applications where the boundary between static and dynamic content often blurs.
Looking forward, this foundation opens several possibilities for enhancement:
-
Integration with translation management systems (TMS) could automate the process of keeping ui.ts up to date, while maintaining type safety through code generation.
-
The route translation system could be extended to handle more complex patterns, such as nested dynamic routes or optional parameters, while preserving its type-safe nature.
-
Performance optimizations could be implemented through strategic code splitting of translation data, loading only the languages needed for each user session.
-
The type system could be further enhanced to support nested translation structures and more sophisticated fallback mechanisms.
Most importantly, this implementation demonstrates how TypeScript’s type system can be leveraged not just for error prevention, but as a tool for building better developer experiences. The combination of compile-time safety and runtime flexibility creates a system that is both reliable and practical for real-world applications.
Through careful attention to type safety, error handling, and framework integration, we’ve created more than just a translation system – we’ve built a foundation for creating truly multilingual applications that developers can work with confidently and users can rely on consistently.
For teams considering a similar implementation, remember that the true value of type safety extends beyond catching errors. It creates a self-documenting codebase where the types themselves serve as living documentation of the system’s capabilities and constraints. This becomes increasingly valuable as applications grow and team members change.
In the end, a well-implemented i18n solution should feel almost invisible to both developers and users. It should guide developers toward correct usage without getting in their way, while providing users with a seamless experience regardless of their chosen language. Through the combination of TypeScript’s type system, careful error handling, and thoughtful API design, we’ve achieved exactly that.