The Psychology of Testing: Moving Beyond 'Should' to 'Want'
· 14m ·
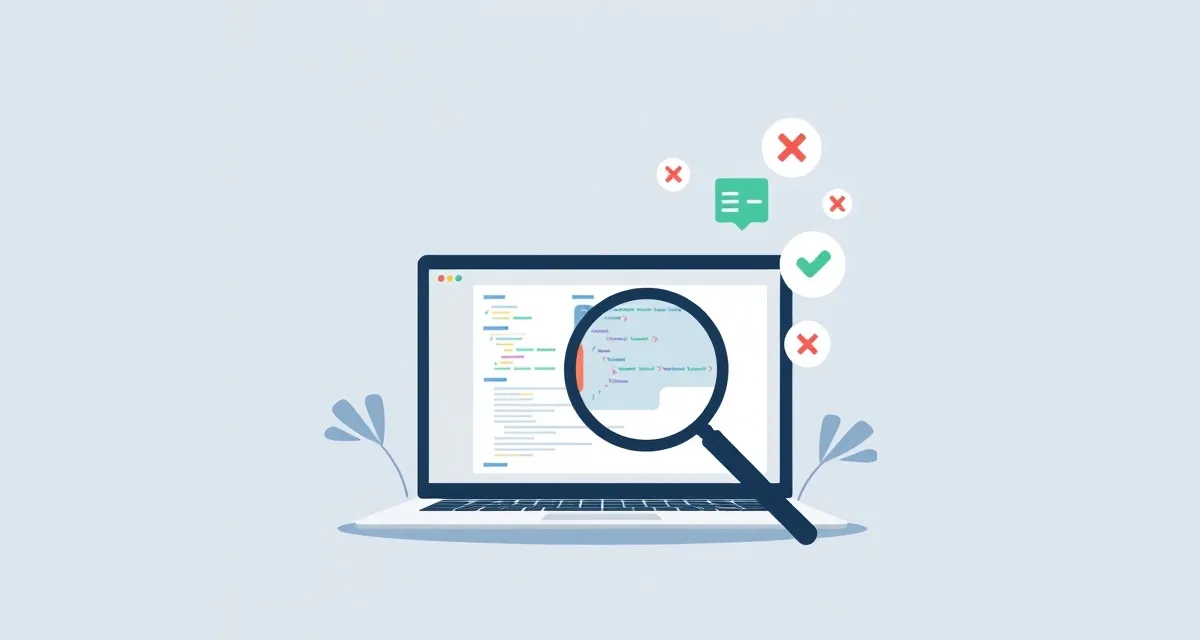
Introduction
Software testing often falls into the category of things developers know they should do but frequently avoid. While most developers acknowledge testing’s importance for code quality, maintainability, and team collaboration, there remains a persistent gap between this acknowledgment and actual implementation. This article explores why this gap exists and, more importantly, how to bridge it by making testing the natural, preferred approach to software development.
The Psychology of Resistance
When asked why they don’t test, developers often cite various reasons: lack of time, complex legacy codebases, or the perception that testing slows down development. However, these explanations often mask the fundamental issue: many developers simply don’t know how to test effectively. Unlike other technical skills where developers freely admit their knowledge gaps, testing carries an implied professional obligation that makes such admissions uncomfortable.
Understanding this psychological barrier is crucial because it shifts our approach from moral imperatives (“you should test”) to practical solutions (“let’s make testing easier than not testing”). This shift in perspective transforms testing from a burden into a natural extension of the development process.
The Path of Least Resistance
The key insight into effective testing isn’t about conviction or discipline – it’s about laziness. As counterintuitive as it might seem, embracing developers’ natural inclination toward efficiency can make testing more appealing. The goal isn’t to force developers to test through willpower or policy, but to make testing the easiest path forward.
Consider a typical debugging scenario without tests:
- Launch the application
- Navigate to the relevant page
- Log in with test credentials
- Reproduce the issue manually
- Make code changes
- Repeat the entire process
This manual approach quickly becomes tedious and time-consuming. In contrast, a well-structured test can:
- Immediately reproduce the issue
- Provide quick feedback on changes
- Enable debugging in isolation
- Maintain consistent test conditions
Design for Testability
The most crucial aspect of testing isn’t writing tests – it’s designing code that’s testable. This distinction is fundamental because it shifts testing from an afterthought to a core design principle. Testable code exhibits several key characteristics:
// Example of code designed for testability
class OrderProcessor {
constructor(
private inventory: InventoryService,
private payment: PaymentService,
private notification: NotificationService
) {}
async processOrder(order: Order): Promise<OrderResult> {
// Each step is isolated and testable
const stockAvailable = await this.inventory.checkStock(order);
if (!stockAvailable) {
return { status: 'failed', reason: 'out_of_stock' };
}
const paymentResult = await this.payment.process(order.total);
if (!paymentResult.success) {
return { status: 'failed', reason: 'payment_declined' };
}
await this.inventory.reserve(order);
await this.notification.sendConfirmation(order);
return { status: 'completed', orderId: order.id };
}
}
This example demonstrates several testability principles:
- Dependencies are explicit and injectable
- Each step has a clear purpose and can be tested in isolation
- The flow is linear and predictable
- The code returns clear results that can be verified
The Evolution of QA’s Role
The traditional view of QA as manual testers clicking through applications is evolving into a more sophisticated and technical role. Modern QA professionals are increasingly focused on:
- Building Testing Infrastructure
// Example of a QA-developed testing utility
class TestEnvironment {
async setup() {
const testDb = await TestDatabase.create();
const mockServices = await MockServiceFactory.create();
return {
database: testDb,
services: mockServices,
cleanup: async () => {
await testDb.teardown();
await mockServices.stop();
}
};
}
}
- Creating Domain-Specific Testing Languages
// Example of a QA-designed test helper
class OrderTestBuilder {
private order: Order = new Order();
withProducts(products: Product[]) {
products.forEach(p => this.order.addProduct(p));
return this;
}
withShippingAddress(address: Address) {
this.order.setShippingAddress(address);
return this;
}
build() {
return this.order;
}
}
These examples show how QA’s role has evolved from verification to enablement, helping developers create more testable code and more effective tests.
The Test-Driven Mindset
While Test-Driven Development (TDD) is a powerful approach, it’s important to be honest about its practical challenges. Even experienced practitioners don’t always follow TDD strictly. This honesty helps teams adopt a more realistic and sustainable approach to testing.
// Example of pragmatic TDD
describe('ShoppingCart', () => {
it('should apply bulk discount when applicable', () => {
// Start with the simplest test case
const cart = new ShoppingCart();
cart.addItem({ id: 'WIDGET', price: 10, quantity: 5 });
// Assert the expected behavior
expect(cart.getTotal()).toBe(45); // 10% off for 5+ items
});
it('should handle mixed quantities correctly', () => {
// Add complexity incrementally
const cart = new ShoppingCart();
cart.addItem({ id: 'WIDGET', price: 10, quantity: 2 });
cart.addItem({ id: 'GADGET', price: 20, quantity: 3 });
expect(cart.getTotal()).toBe(80); // No discount for mixed items < 5
});
});
This approach demonstrates:
- Starting with simple cases
- Incrementally adding complexity
- Building up functionality through tests
- Maintaining clear test intentions
Building a Testing Culture
Creating a sustainable testing culture requires more than technical solutions. It requires fostering an environment where testing is valued and supported. Key elements include:
- Making testing infrastructure a priority
- Celebrating test coverage improvements
- Sharing testing knowledge across the team
- Recognizing testing as a design activity
// Example of a team-focused test
describe('Bug #1234: Order calculation edge case', () => {
it('should handle currency conversion correctly', () => {
// Descriptive test that serves as documentation
const order = new Order();
order.addItem({ price: 10, currency: 'EUR' });
order.addItem({ price: 15, currency: 'USD' });
// Clear assertion with business context
expect(order.getTotalInUSD()).toBe(25.20,
'EUR/USD conversion should use daily exchange rate');
});
});
This test demonstrates how testing can improve team communication by:
- Documenting issues clearly
- Providing reproducible scenarios
- Explaining business logic
- Serving as a reference for future changes
Conclusion
Effective testing isn’t about willpower or discipline – it’s about creating an environment where testing is the most efficient path forward. By focusing on testability in design, leveraging modern tools and practices, and understanding the psychological aspects of developer resistance, we can create a development culture where testing isn’t just something we should do, but something we want to do.
The key is to stop treating testing as a moral imperative and start treating it as a practical tool that makes development easier, faster, and more enjoyable. When testing becomes the path of least resistance, developers naturally choose it not because they should, but because it’s the most efficient way to work.